Python Exception Handling
In this Python exception handling tutorial, we’ll explore how to gracefully manage errors in your Python applications. In the world of programming, errors and exceptions are inevitable. Whether you’re a beginner or an experienced developer, handling exceptions gracefully is a vital skill that ensures your Python applications run smoothly even when unexpected events occur. This blog post dives deep into Python’s exception handling mechanisms, complete with code examples to illustrate each concept.
“If you’re new to Python, check out our Beginner’s Guide to Python.”
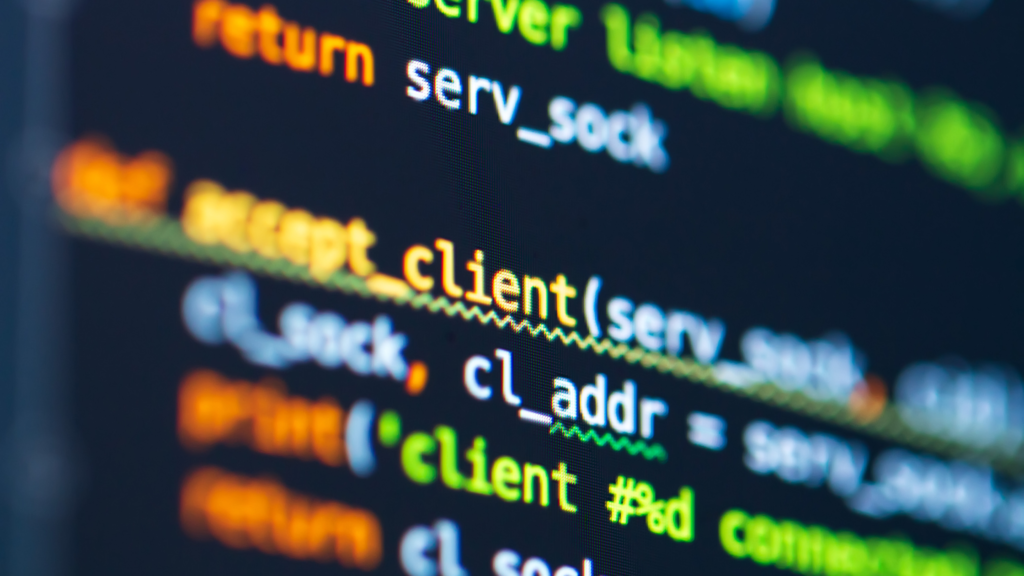
What Are Exceptions?
Exceptions are errors detected during the execution of a program. Unlike syntax errors, which are caught during the compilation phase, exceptions occur when the program is running. For instance:
# Example of an exception number = int("abc") # Raises a ValueError
In the above example, attempting to convert a non-numeric string to an integer raises a ValueError
.
Why Learn Python Exception Handling?
Uncaught exceptions can cause your program to crash, leading to a poor user experience. Exception handling allows you to manage errors gracefully, providing informative feedback to users or retrying operations as needed.
Basic Syntax of Exception Handling
Python provides the try-except
block to handle exceptions. Here’s the general syntax: “For more details, refer to Python’s Official Documentation on Exceptions.”
try: # Code that might raise an exception except ExceptionType: # Code to handle the exception
Example:
try: result = 10 / 0 except ZeroDivisionError: print("You can't divide by zero!")
Output:
You can't divide by zero!
Catching Multiple Exceptions in Python Exception Handling
Sometimes, a block of code might raise different types of exceptions. You can handle them separately:
try: value = int(input("Enter a number: ")) result = 10 / value except ValueError: print("Invalid input! Please enter a number.") except ZeroDivisionError: print("You can't divide by zero!")
Using else
with try-except
The else
block runs if no exceptions are raised in the try
block:
try: result = 10 / 2 except ZeroDivisionError: print("You can't divide by zero!") else: print(f"Result is {result}")
Output:
Result is 5.0
Using finally
in Python Exception Handling
The finally
block runs regardless of whether an exception was raised or not. It’s commonly used for cleanup actions:
try: file = open("example.txt", "r") content = file.read() except FileNotFoundError: print("File not found!") finally: print("Execution complete.")
Output (if file is missing):
File not found! Execution complete.
Raising Exceptions
You can raise exceptions deliberately using the raise
keyword:
def check_age(age): if age < 18: raise ValueError("Age must be 18 or older.") return "Access granted." try: print(check_age(16)) except ValueError as e: print(e)
Output:
Age must be 18 or older.
Custom Exceptions
Python allows you to define your own exceptions by creating classes that inherit from the Exception
class:
class CustomError(Exception): pass try: raise CustomError("This is a custom error message.") except CustomError as e: print(e)
Output:
This is a custom error message.
Best Practices for Exception Handling
- Be Specific: Catch only the exceptions you expect and can handle.
try: # Risky code except (ValueError, KeyError): # Handle specific exceptions
- Avoid Bare
except
: Catching all exceptions without specifying the type can mask unexpected errors.try: # Risky code except Exception as e: print(f"An error occurred: {e}")
- Clean Up Resources: Use
finally
or context managers (e.g.,with
statements) to ensure resources are released properly. - Don’t Ignore Exceptions: Handle them appropriately or re-raise them if necessary.
try: # Risky code except Exception: raise # Re-raise the exception after logging
Advanced Topics
Logging Exceptions
Use the logging
module to log exceptions instead of printing them:
import logging try: result = 10 / 0 except ZeroDivisionError: logging.error("Division by zero occurred", exc_info=True)
Chained Exceptions
Python allows chaining exceptions using the raise ... from ...
syntax:
try: raise ValueError("Initial error") except ValueError as e: raise RuntimeError("Secondary error") from e
Conclusion
Exception handling is a powerful feature in Python that ensures your programs are robust and user-friendly. By mastering the concepts outlined in this guide, you can write code that not only performs well but also gracefully handles unexpected situations. Remember, proper exception handling is not just about preventing crashes—it’s about maintaining the integrity and reliability of your application.