Basic Python Exercises with Solutions (For Beginners)
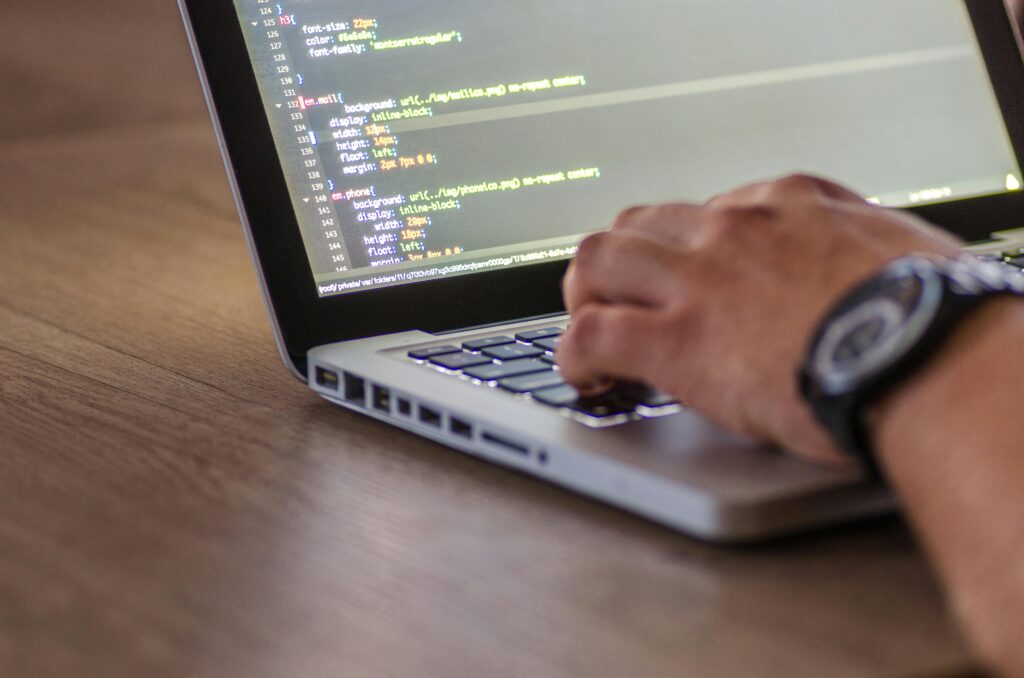
Practicing Python programming through hands-on exercises is one of the most effective ways to learn the language. The following set of beginner-level problems covers fundamental Python concepts such as variables, conditionals, loops, functions, and string manipulation.
Each exercise includes a brief problem statement followed by a complete solution to help reinforce understanding.
✅ Exercise 1: Print "Hello, World!"
Problem:
Write a program to print Hello, World!
.
print("Hello World")
✅ Exercise 1: Add Two Numbers
Problem:
Write a program to add two numbers and print the result.
a = 5
b = 7
sum = a + b
print("Sum:", sum)
Basic Python Exercises with Solutions (For Beginners)
Practicing Python programming through hands-on exercises is one of the most effective ways to learn the language. The following set of beginner-level problems covers fundamental Python concepts such as variables, conditionals, loops, functions, and string manipulation.
Each exercise includes a brief problem statement followed by a complete solution to help reinforce understanding.
✅Exercise 1: Print “Hello, World!”
Problem:
Write a program to print Hello, World!
.
print("Hello, World!")
✅ Exercise 2: Add Two Numbers
Problem:
Write a program to add two numbers and print the result.
a = 5 b = 7 sum = a + b print("Sum:", sum)
✅ Exercise 3: Find the Square of a Number
Problem:
Create a function that returns the square of a number.
def square(n): return n ** 2 print(square(4)) # Output: 16
✅ Exercise 4: Swap Two Variables
Problem:
Swap the values of two variables without using a third variable.
a = 10 b = 20 a, b = b, a print("a =", a) print("b =", b)
✅ Exercise 5: Check Even or Odd
Problem:
Write a program to determine if a number is even or odd.
num = 7 if num % 2 == 0: print("Even") else: print("Odd")
✅ Exercise 6: Find Maximum of Three Numbers
Problem:
Write a function that returns the largest of three numbers.
def maximum(a, b, c): return max(a, b, c) print(maximum(10, 25, 15)) # Output: 25
✅ Exercise 7: Print Multiplication Table
Problem:
Generate and display the multiplication table of a given number.
num = 5 for i in range(1, 11): print(f"{num} x {i} = {num * i}")
✅ Exercise 8: Check for Prime Number
Problem:
Create a function to determine whether a number is prime.
def is_prime(n): if n <= 1: return False for i in range(2, int(n**0.5) + 1): if n % i == 0: return False return True print(is_prime(7)) # Output: True
✅ Exercise 9: Reverse a String
Problem:
Write a function that returns the reverse of a given string.
pythonCopyEdit# Solution
def reverse_string(s):
return s[::-1]
print(reverse_string("python")) # Output: nohtyp
✅ Exercise 10: Count Vowels in a String
Problem:
Write a function to count the number of vowels in a string.
pythonCopyEdit# Solution
def count_vowels(s):
vowels = 'aeiouAEIOU'
count = 0
for char in s:
if char in vowels:
count += 1
return count
print(count_vowels("Hello World")) # Output: 3
📌 Conclusion
These exercises serve as an excellent starting point for Python programming. Practicing such problems helps reinforce basic syntax and logical thinking. After mastering these, it’s beneficial to explore more complex topics such as lists, dictionaries, object-oriented programming, and file handling.
Stay consistent, keep experimenting, and continue building coding confidence through regular practice.