Functions and modules are essential building blocks in Python programming, enabling you to write reusable, modular, and organized code. In this blog post, we’ll explore the basics of functions and modules, how to create them, and their practical applications.
Understanding Python Functions
A function is a block of reusable code that performs a specific task. Functions help to break down complex problems into smaller, manageable parts and promote code reuse.
Creating a Function To define a function in Python, use the def
keyword followed by the function name and parentheses ()
.
Example
def greet(): print("Hello, welcome to LearnProCode!")
Calling a Function To execute a function, simply call it by its name followed by parentheses.
Example:
greet() # Output: Hello, welcome to LearnProCode!
Functions with Parameters Functions can accept parameters (arguments) to provide additional information.
Example:
def greet(name): print(f"Hello, {name}! Welcome to LearnProCode!") greet("Alice") # Output: Hello, Alice! Welcome to LearnProCode!
Functions with Return Values Functions can return values using the return
keyword.
Example:
def add(a, b): return a + b result = add(5, 3) print(result) # Output: 8
Exploring Default Parameters and Lambda Functions in Python
In this blog post, we will delve into two powerful features of Python: default parameters and lambda functions. These features can significantly enhance the flexibility and readability of your code. learn more about python operators.
Default Parameters in Python Function
Default parameters allow you to define a function with default values for one or more parameters. If a value is not provided for a parameter, the default value is used. This feature is useful for creating functions with optional parameters.
Example:
def greet(name, message="Hello"): print(f"{message}, {name}!") # Calling the function with both arguments greet("Alice", "Hi") # Output: Hi, Alice! # Calling the function with only the name argument greet("Bob") # Output: Hello, Bob!
In the example above, the message
parameter has a default value of "Hello"
. When calling greet("Bob")
, the default message is used.
Using Default Parameters in Practice
Example: Creating a User Profile
def create_user_profile(username, age=18, country="USA"): profile = { "username": username, "age": age, "country": country } return profile # Creating profiles with different parameters profile1 = create_user_profile("john_doe") profile2 = create_user_profile("jane_doe", 25, "Canada") print(profile1) # Output: {'username': 'john_doe', 'age': 18, 'country': 'USA'} print(profile2) # Output: {'username': 'jane_doe', 'age': 25, 'country': 'Canada'}
Lambda Functions
Lambda functions, also known as anonymous functions, are small, unnamed functions defined using the lambda
keyword. They are often used for short, simple operations and are written in a single line.
Syntax:
lambda arguments: expression
Example:
# A simple lambda function to add two numbers add = lambda x, y: x + y # Using the lambda function result = add(5, 3) print(result) # Output: 8
Lambda functions are particularly useful when you need a simple function for a short period and do not want to define a full function using def
.
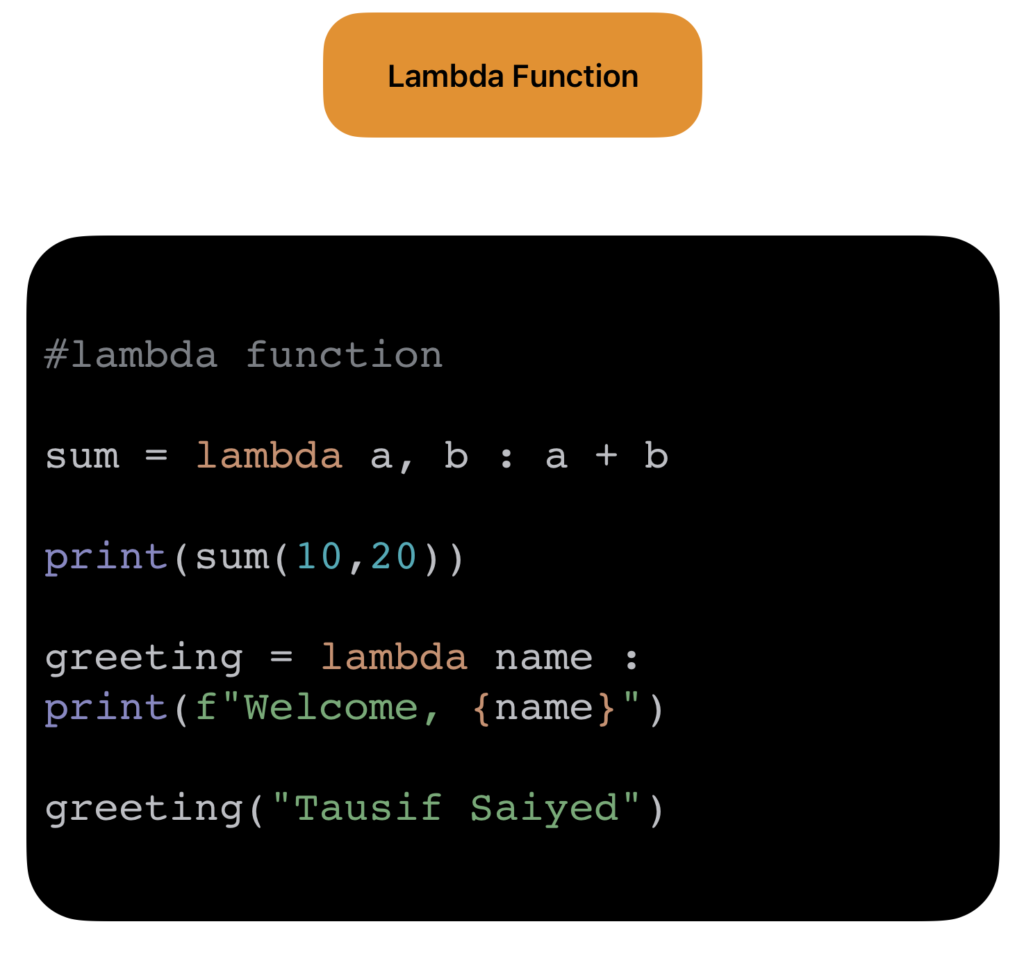
Using Lambda Functions in Practice
Example: Sorting a List of Tuples
# List of tuples students = [("Alice", 25), ("Bob", 20), ("Charlie", 23)] # Sorting by the second element (age) using a lambda function students_sorted = sorted(students, key=lambda student: student[1]) print(students_sorted) # Output: [('Bob', 20), ('Charlie', 23), ('Alice', 25)]
Example: Filtering a List
# List of numbers numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Using a lambda function to filter even numbers even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4, 6, 8, 10]
Example: Mapping a Function to a List
# List of numbers numbers = [1, 2, 3, 4, 5] # Using a lambda function to square each number squared_numbers = list(map(lambda x: x ** 2, numbers)) print(squared_numbers) # Output: [1, 4, 9, 16, 25]
Combining Default Parameters and Lambda Functions
You can also use default parameters and lambda functions together to create flexible and concise code.
Example: Flexible Greeting Function
# Lambda function with a default parameter greet = lambda name, message="Hello": f"{message}, {name}!" # Using the lambda function print(greet("Alice")) # Output: Hello, Alice! print(greet("Bob", "Hi")) # Output: Hi, Bob!
Default parameters and lambda functions are powerful features in Python that can make your code more flexible, concise, and readable. By understanding and utilizing these features, you can write more efficient and elegant code. Keep experimenting with these concepts to see how they can enhance your Python programming skills.
Understanding Multiple Return Functions in Python
In Python, functions can return multiple values using tuples, lists, or dictionaries. This feature is particularly useful when you need to obtain more than one result from a single function call. In this blog post, we will explore how to create and use functions that return multiple values.
Returning Multiple Values Using Tuples
One of the simplest ways to return multiple values from a function is by using a tuple. When you separate values by commas in the return statement, Python automatically packs them into a tuple.
Learn more about tuples here.
Example:
def calculate_statistics(numbers): total = sum(numbers) average = total / len(numbers) return total, average # Calling the function and unpacking the returned tuple numbers = [10, 20, 30, 40, 50] total, average = calculate_statistics(numbers) print(f"Total: {total}, Average: {average}") # Output: Total: 150, Average: 30.0
In the example above, the calculate_statistics
function returns both the total and the average of a list of numbers.
Returning Multiple Values Using Lists
You can also return multiple values as a list. This approach is useful when the number of returned values might change or if you prefer working with lists.
Learn more about Lists here.
Example:
def calculate_min_max(numbers): minimum = min(numbers) maximum = max(numbers) return [minimum, maximum] # Calling the function and unpacking the returned list numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5] min_max = calculate_min_max(numbers) print(f"Minimum: {min_max[0]}, Maximum: {min_max[1]}") # Output: Minimum: 1, Maximum: 9
Returning Multiple Values Using Dictionaries
Using a dictionary to return multiple values can make your code more readable by providing meaningful keys for each returned value. Learn more about dictionaries.
Example:
def calculate_details(numbers): details = { "total": sum(numbers), "average": sum(numbers) / len(numbers), "minimum": min(numbers), "maximum": max(numbers) } return details # Calling the function and accessing the returned dictionary numbers = [5, 8, 2, 9, 4, 7] details = calculate_details(numbers) print(f"Total: {details['total']}, Average: {details['average']}") print(f"Minimum: {details['minimum']}, Maximum: {details['maximum']}") # Output: # Total: 35, Average: 5.833333333333333 # Minimum: 2, Maximum: 9
Practical Examples of Multiple Return Functions
Example 1: Splitting a Full Name
def split_full_name(full_name): first_name, last_name = full_name.split() return first_name, last_name # Calling the function and unpacking the returned tuple full_name = "John Doe" first_name, last_name = split_full_name(full_name) print(f"First Name: {first_name}, Last Name: {last_name}") # Output: First Name: John, Last Name: Doe
Example 2: Calculating Distance and Time
def calculate_distance_time(speed, time): distance = speed * time return distance, time # Calling the function and unpacking the returned tuple speed = 60 # km/h time = 2 # hours distance, time_taken = calculate_distance_time(speed, time) print(f"Distance: {distance} km, Time: {time_taken} hours") # Output: Distance: 120 km, Time: 2 hours
Example 3: Parsing a URL
def parse_url(url): protocol, domain = url.split("://") return protocol, domain # Calling the function and unpacking the returned tuple url = "https://www.example.com" protocol, domain = parse_url(url) print(f"Protocol: {protocol}, Domain: {domain}") # Output: Protocol: https, Domain: www.example.com
Functions that return multiple values are a powerful feature in Python, providing flexibility and convenience in many scenarios. Whether you use tuples, lists, or dictionaries, returning multiple values can help you write cleaner, more organized code. Experiment with these techniques to see how they can enhance your Python programming skills.
Understanding Python Modules
A module is a file containing Python code, which can include functions, classes, and variables. Modules allow you to organize your code into separate files for better manageability and reuse.
Creating a Module To create a module, simply save your Python code in a file with a .py
extension.
Example (mymodule.py
):
def greet(name): print(f"Hello, {name}! Welcome to LearnProCode!") def add(a, b): return a + b
Importing a Module To use a module in another Python file, use the import
statement.
Example (main.py
):
import mymodule mymodule.greet("Alice") # Output: Hello, Alice! Welcome to LearnProCode! result = mymodule.add(5, 3) print(result) # Output: 8
Importing Specific Functions You can import specific functions from a module using the from
keyword.
Example:
from mymodule import greet, add greet("Bob") # Output: Hello, Bob! Welcome to LearnProCode! result = add(10, 7) print(result) # Output: 17
Practical Applications of Functions and Modules
Example 1: Calculator Module
Create a module named calculator.py
with basic arithmetic functions.
# calculator.py def add(a, b): return a + b def subtract(a, b): return a - b def multiply(a, b): return a * b def divide(a, b): if b != 0: return a / b else: return "Error! Division by zero."
Use the calculator
module in another file.
Example 2: Utility Functions
# main.py import calculator print(calculator.add(10, 5)) # Output: 15 print(calculator.subtract(10, 5)) # Output: 5 print(calculator.multiply(10, 5)) # Output: 50 print(calculator.divide(10, 5)) # Output: 2.0
Create a module named utils.py
with various utility functions.
# utils.py def is_even(number): return number % 2 == 0 def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
Use the utils
module in another file.
# main.py import utils print(utils.is_even(10)) # Output: True print(utils.factorial(5)) # Output: 120
Example 3: String Utilities
Create a module named string_utils.py
with functions for string manipulation.
# string_utils.py def to_uppercase(s): return s.upper() def to_lowercase(s): return s.lower()
Use the string_utils
module in another file.
# main.py import string_utils print(string_utils.to_uppercase("hello")) # Output: HELLO print(string_utils.to_lowercase("WORLD")) # Output: world
Conclusion
Functions and modules are vital components of Python programming, enabling you to write clean, efficient, and maintainable code. By mastering these concepts, you can create reusable code blocks and organize your projects effectively. Keep practicing and exploring new ways to utilize functions and modules in your Python projects.